2016-10-18 improve the jquery-ui autocomplete look and feel¶
See also
Last look¶
(django_test_autocomplete_35_64) C:\projects_id3\django-test-autocomplete\projet_ajax>python manage.py runserver 127.0.0.1:8004 Performing system checks...
System check identified no issues (0 silenced).
October 19, 2016 - 09:08:19
Django version 1.10.2, using settings 'projet_ajax.settings'
Starting development server at http://127.0.0.1:8004/
Quit the server with CTRL-BREAK.
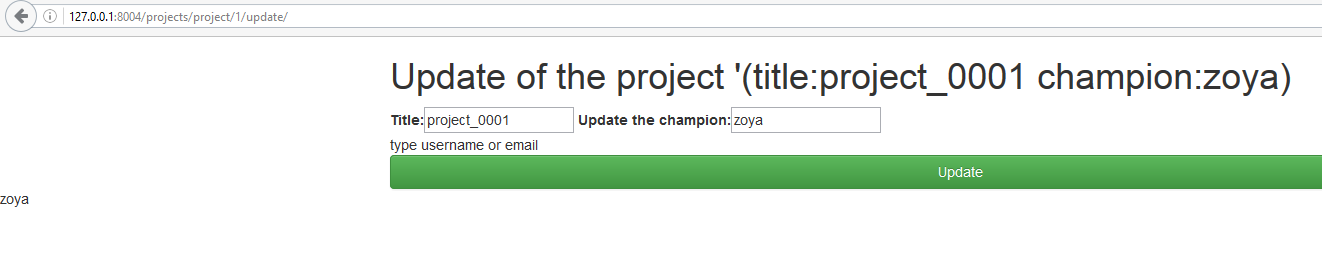
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 | {% extends "base.html" %}
{% load static %}
{% load staticfiles %}
{# STYLE CSS #}
{% block stylesheet %}
{{ block.super }}
{# https://www.bootstrapcdn.com/ #}
<link rel="stylesheet" href="http://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.csss">
<style>
{# https://jqueryui.com/autocomplete/#maxheight #}
.ui-autocomplete {
max-height: 100px;
overflow-y: auto;
/* prevent horizontal scrollbar */
overflow-x: hidden;
}
* html .ui-autocomplete {
height: 100px;
}
</style>
{% endblock %}
{% block content %}
<h1>Update of the project '(title:{{ project.title }} champion:{{ project.champion }}) </h1>
<p></p>
<p></p>
{# https://docs.djangoproject.com/en/dev/topics/forms/ #}
<form id="id_form_project_update" action="{% url 'projects:project_update' project.id %}" method="post">
{% csrf_token %}
<div class="forms">
{{ form.id }}
{{ form.non_field_errors }}
{# Include the hidden fields #}
{% for hidden in form.hidden_fields %}
{{ hidden }}
{% endfor %}
<table id="id_table" class="table table-hover table-bordered table-condensed">
<tbody>
<tr>
<td class="text-right">Title:</td>
<td>{{ form.title }}</td>
</tr>
<tr>
<td class="text-right">Champion:</td>
<td> <span id="id_champion_display">{{ project.champion }}</span> {{ form.champion_term }} </td>
</tr>
</tbody>
</table>
</div>
<input type="submit" name="btn_update" value="Update" class="btn btn-success btn-block" />
</form>
{% if messages %}
<ul class="messages">
{% comment %} https://getbootstrap.com/components/#alerts {% endcomment %}
{% for message in messages %}
<!--li{% if message.tags %} class="{{ message.tags }}"{% endif %} -->
{% if message.level == DEFAULT_MESSAGE_LEVELS.ERROR %}
<div class="alert alert-danger" role="alert">{{ message }}</div>
{% elif message.level == DEFAULT_MESSAGE_LEVELS.SUCCESS %}
<div class="alert alert-success" role="alert">{{ message }}</div>
{% else %}
<div class="alert alert-info" role="alert">{{ message }}</div>
{% endif %}
<!-- /li -->
{% endfor %}
</ul>
{% endif %}
{% endblock content %}
{# BEHAVIOR Javascript #}
{% block javascript %}
{{ block.super }}
{# http://code.jquery.com/ui/ #}
<script src="http://code.jquery.com/ui/1.12.1/jquery-ui.min.js"></script>
{% endblock javascript %}
{# DOM ready #}
{% block domready %}
$( "#id_champion_term" ).autocomplete({
{# http://api.jqueryui.com/autocomplete/#option-source #}
source: "{% url 'projects:champion_get_json' %}",
{# http://api.jqueryui.com/autocomplete/#option-autoFocus #}
autoFocus:true,
{# http://api.jqueryui.com/autocomplete/#option-minLength #}
minLength:1,
{# http://api.jqueryui.com/autocomplete/#event-select #}
select:function(event,ui) {
$("#id_champion").val(ui.item.id);
}
});
{% endblock %}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | #!/usr/bin/python
# -*- coding: utf8 -*-
"""The project's forms.
"""
from django import forms
from .models import Project
class ProjectChampionForm(forms.ModelForm):
"""The champion project form"""
champions_choice_list = forms.CharField(max_length=100,
help_text='type username or email')
class Meta:
model = Project
fields = ('title',
'champions_choice_list', 'champion',)
def __init__(self, *args, **kwargs):
super(ProjectChampionForm, self).__init__(*args, **kwargs)
self.fields['champions_choice_list'].label = "Update the champion"
self.fields['champion'].widget = forms.HiddenInput()
|
jquery-ui autocomplete options already used¶
Remote option¶
The Autocomplete widgets provides suggestions while you type into the field. Here the suggestions are bird names, displayed when at least two characters are entered into the field.
The datasource is a server-side script which returns JSON data, specified via a simple URL for the source-option. In addition, the minLength-option is set to 2 to avoid queries that would return too many results and the select-event is used to display some feedback.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>jQuery UI Autocomplete - Remote datasource</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<style>
.ui-autocomplete-loading {
background: white url("images/ui-anim_basic_16x16.gif") right center no-repeat;
}
</style>
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$( function() {
function log( message ) {
$( "<div>" ).text( message ).prependTo( "#log" );
$( "#log" ).scrollTop( 0 );
}
$( "#birds" ).autocomplete({
source: "search.php",
minLength: 2,
select: function( event, ui ) {
log( "Selected: " + ui.item.value + " aka " + ui.item.id );
}
});
} );
</script>
</head>
<body>
<div class="ui-widget">
<label for="birds">Birds: </label>
<input id="birds">
</div>
<div class="ui-widget" style="margin-top:2em; font-family:Arial">
Result:
<div id="log" style="height: 200px; width: 300px; overflow: auto;" class="ui-widget-content"></div>
</div>
</body>
</html>
Try https://jqueryui.com/autocomplete/#maxheight¶
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>jQuery UI Autocomplete - Scrollable results</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<style>
.ui-autocomplete {
max-height: 100px;
overflow-y: auto;
/* prevent horizontal scrollbar */
overflow-x: hidden;
}
/* IE 6 doesn't support max-height
* we use height instead, but this forces the menu to always be this tall
*/
* html .ui-autocomplete {
height: 100px;
}
</style>
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$( function() {
var availableTags = [
"ActionScript",
"AppleScript",
"Asp",
"BASIC",
"C",
"C++",
"Clojure",
"COBOL",
"ColdFusion",
"Erlang",
"Fortran",
"Groovy",
"Haskell",
"Java",
"JavaScript",
"Lisp",
"Perl",
"PHP",
"Python",
"Ruby",
"Scala",
"Scheme"
];
$( "#tags" ).autocomplete({
source: availableTags
});
} );
</script>
</head>
<body>
<div class="ui-widget">
<label for="tags">Tags: </label>
<input id="tags">
</div>
</body>
</html>
Try https://jqueryui.com/autocomplete/#categories¶
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>jQuery UI Autocomplete - Categories</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<style>
.ui-autocomplete-category {
font-weight: bold;
padding: .2em .4em;
margin: .8em 0 .2em;
line-height: 1.5;
}
</style>
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$( function() {
$.widget( "custom.catcomplete", $.ui.autocomplete, {
_create: function() {
this._super();
this.widget().menu( "option", "items", "> :not(.ui-autocomplete-category)" );
},
_renderMenu: function( ul, items ) {
var that = this,
currentCategory = "";
$.each( items, function( index, item ) {
var li;
if ( item.category != currentCategory ) {
ul.append( "<li class='ui-autocomplete-category'>" + item.category + "</li>" );
currentCategory = item.category;
}
li = that._renderItemData( ul, item );
if ( item.category ) {
li.attr( "aria-label", item.category + " : " + item.label );
}
});
}
});
var data = [
{ label: "anders", category: "" },
{ label: "andreas", category: "" },
{ label: "antal", category: "" },
{ label: "annhhx10", category: "Products" },
{ label: "annk K12", category: "Products" },
{ label: "annttop C13", category: "Products" },
{ label: "anders andersson", category: "People" },
{ label: "andreas andersson", category: "People" },
{ label: "andreas johnson", category: "People" }
];
$( "#search" ).catcomplete({
delay: 0,
source: data
});
} );
</script>
</head>
<body>
<label for="search">Search: </label>
<input id="search">
</body>
</html>
Try https://jqueryui.com/autocomplete/#remote-jsonp¶
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>jQuery UI Autocomplete - Remote JSONP datasource</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<style>
.ui-autocomplete-loading {
background: white url("images/ui-anim_basic_16x16.gif") right center no-repeat;
}
</style>
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$( function() {
function log( message ) {
$( "<div>" ).text( message ).prependTo( "#log" );
$( "#log" ).scrollTop( 0 );
}
$( "#birds" ).autocomplete({
source: function( request, response ) {
$.ajax( {
url: "search.php",
dataType: "jsonp",
data: {
term: request.term
},
success: function( data ) {
response( data );
}
} );
},
minLength: 2,
select: function( event, ui ) {
log( "Selected: " + ui.item.value + " aka " + ui.item.id );
}
} );
} );
</script>
</head>
<body>
<div class="ui-widget">
<label for="birds">Birds: </label>
<input id="birds">
</div>
<div class="ui-widget" style="margin-top:2em; font-family:Arial">
Result:
<div id="log" style="height: 200px; width: 300px; overflow: auto;" class="ui-widget-content"></div>
</div>
</body>
</html>